centos 7, apache event MPM with php-fpm
Create GeoIP Badge Using PHP Image Processing And GD
When making the badges I always use javascript. This tutorial shows you how to use the GD library to dynamically create simple badges on your site.
Basically, image generation is done in three steps:
- allocating an image
- drawing into the allocated space
- releasing the allocated data in picture format to the browser
[cfields]badgegd[/cfields]
As usual, obtaining client Ip Address simply by calling this php predefined variable, this time we will also trying to support for IPv6. One drawback, i still don’t know how to resize canvas to be fit with text if overflown, dynamically. Ie: the long IPv6 address.
<?php echo $_SERVER["REMOTE_ADDR"]; ?>
Show GeoIP Badge On Your Website Using PHP And JavaScript
Remember my last article about how to show visitor’s ip address on our website? today, we will modify the script a little bit to do something more interesting, not only displaying visitor’s ip address but also displaying visitor’s country and flag. Unfortunately this script unable displaying the flag and country for ipv6 yet. The badge will look something like this.
[cfields]geoipbadge[/cfields]
First thing we have to do is create badge generator, which we will use later. let’s call it geo.php
<?php if ( isset($_SERVER["REMOTE_ADDR"]) ) { $IpAddr=$_SERVER["REMOTE_ADDR"]; } $cc = geoip_country_code_by_name($IpAddr); $country = geoip_country_name_by_name($IpAddr); ?> if (typeof(v_geo_BackColor)=="undefined") v_geo_BackColor = "white"; if (typeof(v_geo_ForeColor)=="undefined") v_geo_ForeColor= "black"; if (typeof(v_geo_FontPix)=="undefined") v_geo_FontPix = "16"; if (typeof(v_geo_DisplayFormat)=="undefined") v_geo_DisplayFormat = "You are visiting from:<br>IP Address: %%IP%%%%FLAG%% %%COUNTRY%%"; if (typeof(v_geo_DisplayOnPage)=="undefined" || v_geo_DisplayOnPage.toString().toLowerCase()!="no") v_geo_DisplayOnPage = "yes"; v_geo_HostIP = "<?php echo $IpAddr; ?>"; v_geo_Country = "<?php echo $country; ?>"; <?php if ( $cc != FALSE ) { ?> v_geo_Flag = "<br><img src='http://www.example.com/flags/<?php echo strtolower($cc); ?>.png' />"; <?php } else { ?> v_geo_Flag = ""; <?php } ?> if (v_geo_DisplayOnPage=="yes") { v_geo_DisplayFormat = v_geo_DisplayFormat.replace(/%%IP%%/g, v_geo_HostIP); v_geo_DisplayFormat = v_geo_DisplayFormat.replace(/%%FLAG%%/g, v_geo_Flag); v_geo_DisplayFormat = v_geo_DisplayFormat.replace(/%%COUNTRY%%/g, v_geo_Country); document.write("<table border='0' style='padding: 5px; -moz-border-radius: 5px; -webkit-border-radius: 5px; -khtml-border-radius: 5px; border-radius: 5px; background-color:" + v_geo_BackColor + "; color:" + v_geo_ForeColor + "; font-size:" + v_geo_FontPix + "px'><tr><td>" + v_geo_DisplayFormat + "</td></tr></table>"); }
Create Your Own Visitor’s IP Address Badge Using PHP And JavaScript
A web badge is a small image or text used on websites to promote web standards, products used in the creation of a web page or product, to indicate a specific content license that is applied to the content or design of a website, to comply with an application’s terms of service, to encourage your visitors to check your social network status, or even to display visitor’s informations. such as ip address, user agent, hostname etc.
Today’s story, we’ll make a badge for visitor’s ip address using php and javascript. This badge, for an example:
[cfields]v_ip_badge[/cfields]
create a php script called ipaddr.php as follows
<?php if ( isset($_SERVER["REMOTE_ADDR"]) ) { $ip=$_SERVER["REMOTE_ADDR"] . ' '; } else if ( isset($_SERVER["HTTP_X_FORWARDED_FOR"]) ) { $ip=$_SERVER["HTTP_X_FORWARDED_FOR"] . ' '; } else if ( isset($_SERVER["HTTP_CLIENT_IP"]) ) { $ip=$_SERVER["HTTP_CLIENT_IP"] . ' '; } $IpAddr = $ip; ?> if (typeof(v_ip_BackColor)=="undefined") v_ip_BackColor = "white"; if (typeof(v_ip_ForeColor)=="undefined") v_ip_ForeColor= "black"; if (typeof(v_ip_FontPix)=="undefined") v_ip_FontPix = "16"; if (typeof(v_ip_DisplayFormat)=="undefined") v_ip_DisplayFormat = "You are visiting from:<br>IP Address: %%IP%%"; if (typeof(v_ip_DisplayOnPage)=="undefined" || v_ip_DisplayOnPage.toString().toLowerCase()!="no") v_ip_DisplayOnPage = "yes"; v_ip_HostIP = "<?php echo $IpAddr ?>"; if (v_ip_DisplayOnPage=="yes") { v_ip_DisplayFormat = v_ip_DisplayFormat.replace(/%%IP%%/g, v_ip_HostIP); document.write("<table border='0' cellspacing='0' cellpadding='5' style='background-color:" + v_ip_BackColor + "; color:" + v_ip_ForeColor + "; font-size:" + v_ip_FontPix + "px'><tr><td>" + v_ip_DisplayFormat + "</td></tr></table>"); }
Nginx Memcached module And PHP
I’ve seen lots of tutorials on internet explaining how set nginx to use memcached module. But, i’ve rarely seen them explaining, even the easiest one on how to populate memcached with data generated by php. When i’m getting in touch with memcached for the first time, i thought default configuration like this will be working out of the box. without touching php script. 😀
server { listen 80; server_name example.com www.example.com; # ... location ~ \.php$ { set $memcached_key "kutu:$request_uri"; memcached_pass 127.0.0.1:11211; default_type text/html; error_page 404 405 502 = @cache_miss; } location @cache_miss { fastcgi_pass 127.0.0.1:9000; fastcgi_index index.php; fastcgi_param SCRIPT_FILENAME /path/to/yoursite/htdocs$fastcgi_script_name; include /etc/nginx/fastcgi_params; } # ... }
Well, i was totally confused :D. so, to prevent other people misinterpret on how nginx memcached module works, i wrote simple examples, and simple explainations on how to configure nginx and populate data generated from php.
Memcached And PHP, Caching Mysql Query Result
I’ve been messing around with memcached and php-pecl-memcache to cache sql query result. Many web sites & applications such as Facebook, LiveJournal, Flickr, Slashdot, WikiPedia/MediaWiki, SourceForge, Digg and Twitter use memcached to enhance their performance.
Memcached (Memory Cache Daemon) was developed by the team at LiveJournal to improve performance of their social blogging site by minimizing the impact of the bottleneck caused by reading data directly from the database. Memcached is a server that caches Name Value Pairs in memory. The “Name”, or key, is limited to 250 characters, and the “Value” is limited to 1MB in size. Values can consist of data, HTML Fragments, or binary objects; almost any type of data that can be serialized and fits in memcached can be stored.
here is simple example/demonstration how to cache regular sql query
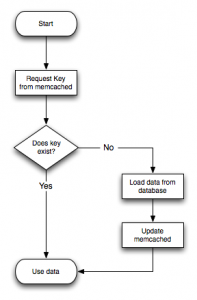
First of all, we need memcached daemon run on system
$ ps ax | grep memcached 8955 ? Ssl 0:00 memcached -d -p 11211 -u memcached -m 256 -c 1024 -P /var/run/memcached/memcached.pid -l 127.0.0.1
Setup simple mysql database/tables as shown bellow:
mysql-shell> CREATE DATABASE memcache;
Copy/Paste this tables schema to your mysql shell/console
CREATE TABLE memc ( personID int NOT NULL AUTO_INCREMENT, PRIMARY KEY(personID), FirstName varchar(15), LastName varchar(15), Age int ); hit enter/return key
Insert some data
mysql-shell> INSERT INTO memc (FirstName, LastName, Age) VALUES('Memory', 'Cache', '100');
How To Make php-fpm Listen On Both Tcp And Unix Socket?
I need to make php-fpm listeing on both tcp/unix socket, and this is how it done.
(this was not pretty workarround i guess, but it work 😀 )
first download php rpm source
$ wget http://centos.alt.ru/pub/php-fpm/5.3.3-2/SRPMS/php-5.3.3-2.el5.src.rpm
Compile and install
$ rpmbuild --rebuild php-5.3.3-2.el5.src.rpm $ sudo rpm -Uvh /path/to/RPMS/php-*
Configuring the default php-fpm for using tcp socket
Edit www.conf
$ sudo vi /etc/php-fpm.d/www.conf
Find line containing
listen = 127.0.0.1:9000
We can make it listening to port what ever we desire, ie 9001 etc
Start php-fpm first instance
$ sudo service php-fpm start
Configuring php-fpm for using unix socket
$ sudo cp /etc/php-fpm.conf /etc/php-fpm2.conf $ sudo cp -rp /etc/php-fpm.d /etc/php-fpm2.d
Edit /etc/php-fpm2.conf
include=/etc/php-fpm2.d/*.conf pid = /var/run/php-fpm/php-fpm2.pid error_log = /var/log/php-fpm/error2.log
Edit /etc/php-fpm2.d/www.conf
listen = /tmp/php-fpm.sock php_admin_value[error_log] = /var/log/php-fpm/www-error2.log
Speeding up your website using eaccelerator and memcache/memcached
Php default behaviour is always re-compile our script everytime accessed by users/browsers. eaccelerator can increases the performance of PHP scripts by caching them in their compiled state, so that the overhead of compiling is almost completely eliminated. It also optimizes scripts to speed up their execution. eAccelerator typically reduces server load and increases the speed of your PHP code by 1-10 times.
memcached is a high-performance, distributed memory object caching system, generic in nature, but intended for use in speeding up dynamic web applications by alleviating database load.
Here’s how to install eaccelerator extension
$ wget http://bart.eaccelerator.net/source/0.9.6/eaccelerator-0.9.6-rc1.tar.bz2 $ tar xjf eaccelerator-0.9.6-rc1.tar.bz2 $ cd eaccelerator-0.9.6-rc1 $ phpize $ ./configure $ make $ sudo make install $ cd /var/cache $ sudo mkdir eaccelerator $ sudo chown -R apache:apache eaccelerator
Hello World
A “hello world” program has become the traditional first program that many people learn. Here’s i’ll show you how to print “Hello World” in A web page.
Using HTML
<!DOCTYPE html PUBLIC "-//IETF//DTD HTML 2.0//EN"> <HTML> <HEAD> <TITLE> Hello World </TITLE> </HEAD> <BODY> <P>hello world</P> </BODY> </HTML>